Swift開婦捕獲用戶簽名是需要抓取用戶手寫或屏幕上的簽名然後生成一張圖片了,有點像把驗證碼生成圖片一樣的原理了,下面我們來看看如何實現。 本文介紹如何使用Swift語言,在iOS設備上捕捉用戶的簽名(其實這個本質就是一個簡單的畫圖板程序)。
實現功能如下:
1,頁面上方提供一個簽名區域(UIView),用戶可以在這個區域手寫簽字。
2,點擊“預覽簽名”,會獲取用戶簽名生成UIImage,在下方的imageView中顯示。
3,點擊“保存簽名”,會將用戶簽名保存到設備相冊中。
效果圖如下:
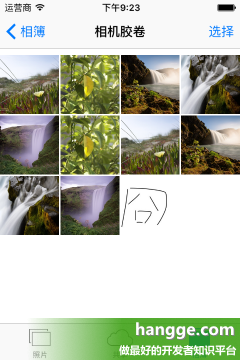
代碼如下:
--- DrawSignatureView.swift (簽名視圖組件) ---
代碼如下
復制代碼
import UIKit
public class DrawSignatureView: UIView {
// 公共屬性
public var lineWidth: CGFloat = 2.0 {
didSet {
self.path.lineWidth = lineWidth
}
}
public var strokeColor: UIColor = UIColor.blackColor()
public var signatureBackgroundColor: UIColor = UIColor.whiteColor()
// 私有屬性
private var path = UIBezierPath()
private var pts = [CGPoint](count: 5, repeatedValue: CGPoint())
private var ctr = 0
// Init
override init(frame: CGRect) {
super.init(frame: frame)
self.backgroundColor = self.signatureBackgroundColor
self.path.lineWidth = self.lineWidth
}
// Init
required public init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
self.backgroundColor = self.signatureBackgroundColor
self.path.lineWidth = self.lineWidth
}
// Draw
override public func drawRect(rect: CGRect) {
self.strokeColor.setStroke()
self.path.stroke()
}
// 觸摸簽名相關方法
override public func touchesBegan(touches: Set<UITouch>, withEvent event: UIEvent?) {
if let firstTouch = touches.first{
let touchPoint = firstTouch.locationInView(self)
self.ctr = 0
self.pts[0] = touchPoint
}
}
override public func touchesMoved(touches: Set<UITouch>, withEvent event: UIEvent?) {
if let firstTouch = touches.first{
let touchPoint = firstTouch.locationInView(self)
self.ctr++
self.pts[self.ctr] = touchPoint
if (self.ctr == 4) {
self.pts[3] = CGPointMake((self.pts[2].x + self.pts[4].x)/2.0,
(self.pts[2].y + self.pts[4].y)/2.0)
self.path.moveToPoint(self.pts[0])
self.path.addCurveToPoint(self.pts[3], controlPoint1:self.pts[1],
controlPoint2:self.pts[2])
self.setNeedsDisplay()
self.pts[0] = self.pts[3]
self.pts[1] = self.pts[4]
self.ctr = 1
}
self.setNeedsDisplay()
}
}
override public func touchesEnded(touches: Set<UITouch>, withEvent event: UIEvent?) {
if self.ctr == 0{
let touchPoint = self.pts[0]
self.path.moveToPoint(CGPointMake(touchPoint.x-1.0,touchPoint.y))
self.path.addLineToPoint(CGPointMake(touchPoint.x+1.0,touchPoint.y))
self.setNeedsDisplay()
} else {
self.ctr = 0
}
}
// 簽名視圖清空
public func clearSignature() {
self.path.removeAllPoints()
self.setNeedsDisplay()
}
// 將簽名保存為UIImage
public func getSignature() ->UIImage {
UIGraphicsBeginImageContext(CGSizeMake(self.bounds.size.width,
self.bounds.size.height))
self.layer.renderInContext(UIGraphicsGetCurrentContext()!)
let signature: UIImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return signature
}
}
--- ViewController.swift (使用樣例) ---
代碼如下
復制代碼
import UIKit
class ViewController: UIViewController {
//簽名預覽
@IBOutlet weak var imageView: UIImageView!
//簽名區域視圖
var drawView:DrawSignatureView!
override func viewDidLoad() {
super.viewDidLoad()
//簽名區域位置尺寸
var drawViewFrame = self.view.bounds
drawViewFrame.size.height = 200
//添加簽名區域
drawView = DrawSignatureView(frame: drawViewFrame)
self.view.addSubview(drawView)
}
//預覽簽名
@IBAction func previewSignature(sender: AnyObject) {
let signatureImage = self.drawView.getSignature()
imageView.image = signatureImage
}
//保存簽名
@IBAction func savaSignature(sender: AnyObject) {
let signatureImage = self.drawView.getSignature()
UIImageWriteToSavedPhotosAlbum(signatureImage, nil, nil, nil)
self.drawView.clearSignature()
}
//清除簽名
@IBAction func clearSignature(sender: AnyObject) {
self.drawView.clearSignature()
self.imageView.image = nil
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
}